Connecting to SharePoint 2007 Web Services using a Service Reference in Visual Studio 2008
22 April, 2009 2 Comments
When using Service References to consume SharePoint web services, there are a few additional configuration changes that you need to make. The service model configuration that Visual Studio generates is not sufficient when consuming SharePoint web services. This is mainly what we will cover in this post.
You may have noticed in Visual Studio 2008 that Web References are no longer the default option when you wish to reference a Web Service. Instead, you now have an option called “Add Service Reference….”
So what’s the difference between a Web Reference and a Service Reference? Well basically, a Web Reference is a wrapper over wsdl.exe and a Service Reference is a wrapper over svcutil.exe. Service references also have some additional configuration options and are a good choice when planning to consume WCF services.
1. First step, create a new project in Visual Studio. I have created a new Windows Forms Application and targeted it at .NET 3.5.
2. Right click on “References” in the solution explorer and select “Add Service Reference”
3. I am going to connect to the Lists service on my local SharePoint instance. My web application URL is http://localhost:120/ so the Address for Lists Web Service will be http://localhost:120/_vti_bin/Lists.asmx.
Enter the URL in the Address section and click Go. Make sure you give the service reference a Namespace. I have used “ListReference” for this demo.
4. Next, let’s create a button and combo box on the form. We will use the button to execute a method that gets a list of SharePoint lists on the site and display them in the combo box.
5. Double click the button and we will add some code to the “click” event to invoke the web service method and get the list of Lists.
First change we need to make is in the binding configuration security element. The automatically generated code produces the following configuration settings:
7. In the button click method, add the following code in the try{..} block after we open the connection to the service.
You may have noticed in Visual Studio 2008 that Web References are no longer the default option when you wish to reference a Web Service. Instead, you now have an option called “Add Service Reference….”
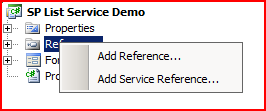
So what’s the difference between a Web Reference and a Service Reference? Well basically, a Web Reference is a wrapper over wsdl.exe and a Service Reference is a wrapper over svcutil.exe. Service references also have some additional configuration options and are a good choice when planning to consume WCF services.
1. First step, create a new project in Visual Studio. I have created a new Windows Forms Application and targeted it at .NET 3.5.
2. Right click on “References” in the solution explorer and select “Add Service Reference”
3. I am going to connect to the Lists service on my local SharePoint instance. My web application URL is http://localhost:120/ so the Address for Lists Web Service will be http://localhost:120/_vti_bin/Lists.asmx.

Enter the URL in the Address section and click Go. Make sure you give the service reference a Namespace. I have used “ListReference” for this demo.
4. Next, let’s create a button and combo box on the form. We will use the button to execute a method that gets a list of SharePoint lists on the site and display them in the combo box.
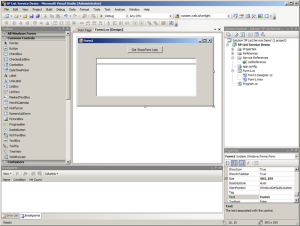
5. Double click the button and we will add some code to the “click” event to invoke the web service method and get the list of Lists.
6. Next things get interesting. We will need to make some changes to the app.config file (or web.config if you in Web Application) as the automatically generated service binding configuration doesn’t work for a SharePoint service. The main changes we will make are around security as we need to enable impersonation and ensure the connection is made using Windows Authentication.1: private void button1_Click(object sender, EventArgs e)2: {3: ListsSoapClient client = null;4:5: try
6: {7: client = new ListsSoapClient();8:9: // Open the connection to the web service
10: client.Open();11:12: // Write some code to query the web service and get a list of
13: // Lists.
14:15:16: }17: catch (Exception ex)18: {19: MessageBox.Show(ex.Message, "An error has occured", MessageBoxButtons.OK, MessageBoxIcon.Error);20: }21: finally
22: {23: client.Close();24: }25: }
First change we need to make is in the binding configuration security element. The automatically generated code produces the following configuration settings:
we will need to change it to the following:1: <security mode="None">2: <transport clientCredentialType="None" proxyCredentialType="None" realm="" />3: <message clientCredentialType="UserName" algorithmSuite="Default" />4: </security>
Next, we will need to add a service behaviour. By default, no service behaviours are generated. This behaviour will enable impersonation.1: <security mode="TransportCredentialOnly">2: <transport clientCredentialType="Ntlm" proxyCredentialType="Ntlm"/>3: </security>
And add a reference to this Behaviour in the endpoint configuration:1: <behaviors>2: <endpointBehaviors>3: <behavior name="ListServiceBehavior">4: <clientCredentials>5: <windows allowedImpersonationLevel="Impersonation"/>6: </clientCredentials>7: </behavior>8: </endpointBehaviors>9: </behaviors>
OK. That’s the service binding configuration sorted. Now back to our code….1: <endpoint address="http://localhost:120/_vti_bin/Lists.asmx"2: binding="basicHttpBinding" bindingConfiguration="ListsSoap"3: contract="ListReference.ListsSoap" name="ListsSoap"4: behaviorConfiguration="ListsServiceBehavior"/>
7. In the button click method, add the following code in the try{..} block after we open the connection to the service.
And there you have it. Run your application, click the button and you should get a list of Lists!1: var response = client.GetListCollection();
2: foreach (XmlNode listNode in response.ChildNodes)3: {4: comboBox1.Items.Add(listNode.Attributes.GetNamedItem("Title").Value);
5: }
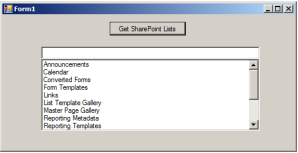
No comments:
Post a Comment